CS 115 Lab 1, Part A: Edit a text-based Python program using IDLE
[Back to Lab 1 instructions]
Summary
In this part of the lab, you will use IDLE to write and run a Python program. The screenshots
on this page were taken on a Mac, but IDLE should work similarly on Windows.
Instructions
- If you haven't done the pre-lab assignment yet, do it first!! You will need to open up the pre-lab instructions in one tab and the Moodle "quiz" in another.
- Open IDLE.
- In Mac OS X... You can find IDLE by clicking on the magnifying glass
at the top right of the screen and typing IDLE.
Click on the top result to open the program.
- In Windows 7... Click the Windows icon at the lower left of the screen
and type IDLE into the search box. Click IDLE (Python GUI) to open
the program.
- In IDLE, go to the File menu and select New File or New Window:
- In the new IDLE window (not the Python Shell window),
type in (or copy-paste) the following program exactly as written:
"""
Lab 1: This program prompts for your name and then greets you by name.
Author: ____________
"""
def main():
name = input('What is your name? ')
print('Hi there,', name)
main()
- Modify the docstring at the top of the program to add yourself as the author of the program.
- Go to the File menu, then select Save As. Save your program as lab01.py in the current folder (which should be C:\Python34 in Windows and the Documents folder in Mac OS):
- Go to the Run menu, then select Run Module:
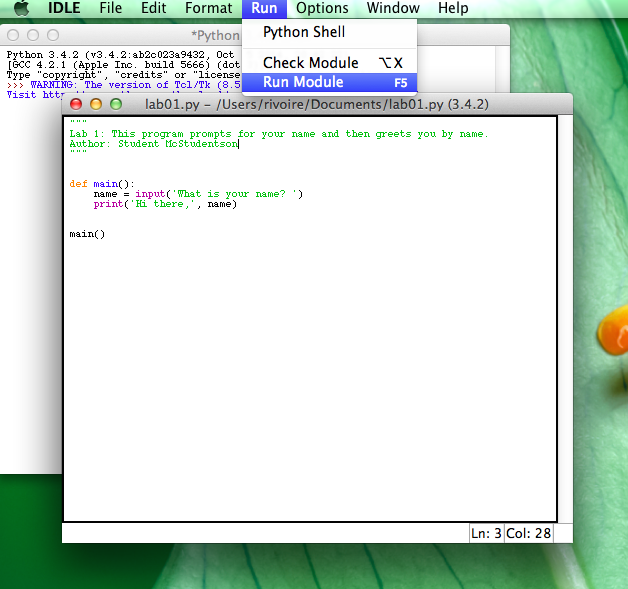
After you select "Run Module," your program will run in the other window
(the "Python Shell" window):
- When you are prompted, type your name and hit the return key.
- Keep the docstring at the top, but modify the rest of your program so that it looks like this:
def main():
name = input('What is your name? ')
print('Hi there, ', name, '.', sep="")
main()
- Run your program again. Notice how the output changed, and be sure you understand why.
- Answer Question 1 in the Lab 1 writeup. Be sure to check your answers.
- Back in your source code window, modify the program so that it asks you for your favorite movie
and then tells you that your taste is terrible.
Here is an example of what your program should do. In this example,
the user's input is
underlined and italicized,
and the rest of the text should be printed by your program:
What is your favorite movie? Transformers
Ugh, Transformers is a terrible movie.
- Here is another example. In this example, the user's input is Mission Impossible.
What is your favorite movie? Mission Impossible
Ugh, Mission Impossible is a terrible movie.
- Change the first line of the docstring so that it accurately describes what the program does now.
- Continue to Part B.